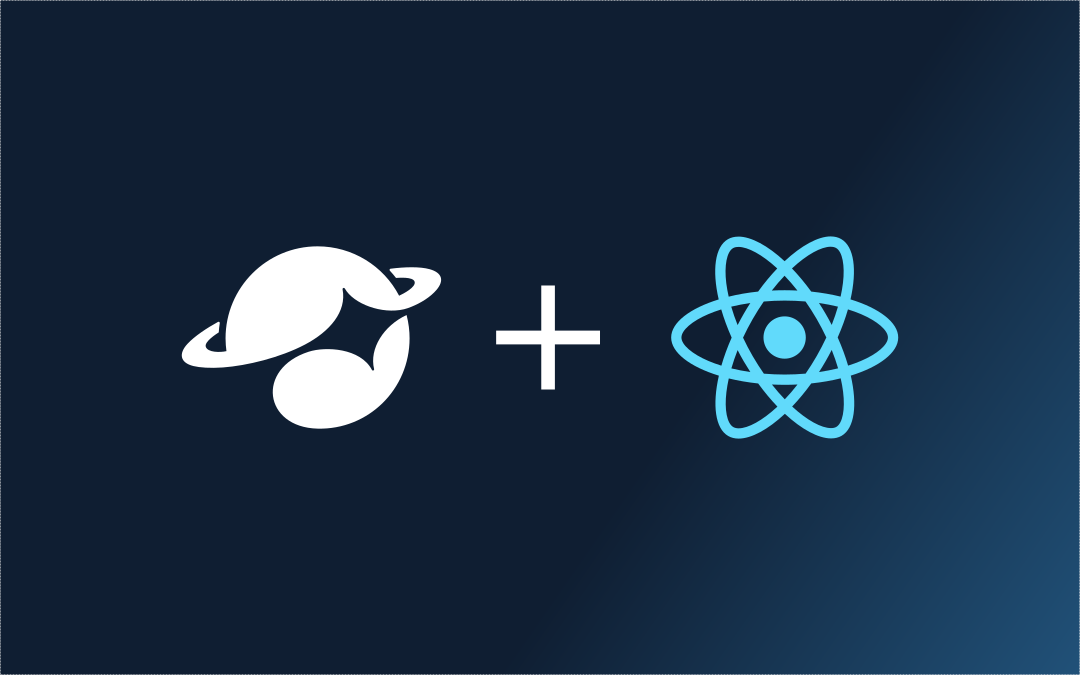
Introduction
While hooks are pretty cool, they can be quite difficult to master. There are a lot of rules and special techniques required to use them correctly (can only be used inside components, cannot be conditionally called, some hooks are sometimes run twice etc). Using them incorrectly can lead to bugs and degraded performance.
Signals
Signals offer a pragmatic alternative to state handling and events. They're performant out of the box, very lightweight, and are easy to use and to reason about.
Let's try a quick example!
First install the React Signals package from Preact:
npm install @preact/signals-react
To show how Signals work in an example, let's create a simple component that renders a button with a small counter in it. In order to showcase some of the functionality of Signals we'll make it a little spicy!
import { effect, signal } from '@preact/signals-react';
// Create our signal and set the original value to `0`.
const count = signal(0);
// Every time the value of the signal changes, log to console. N.B. that we
// didn't specify any dependencies! Signals has all the information it needs.
effect(() => console.log(count.value));
// Every 5 seconds, change the count to a random number -- from outside of the
// component no less!
setInterval(() => {
count.value = Math.random();
}, 5000);
const Counter = () => (
<button onClick={() => count.value++}>{count}</button>
);
export default Counter;
export { count };
If we want to access the signal from another component we can simply import it!
import { count } from "./Counter";
function DisplayCount() {
return (
<div>Count: {count}</div>
);
}
export default DisplayCount;
Super-simple, yet very powerful! You can read more about Signals in this awesome article by Preact.